CCP Stack Plotting#
The stacks computed and saved by the routines have a few built-in plotting tools for simple visualization
Volume Sections#
Here we compute a CCP volume first and subsequently slice through it by simply
taking slices from a 3D matrix using plot_volume_sections()
.
1import numpy as np
2
3from pyglimer.ccp.ccp import read_ccp
4from pyglimer.plot.plot_utils import set_mpl_params
5import matplotlib.pyplot as plt
6set_mpl_params()
7
8# Read the CCP Stack
9ccpstack = read_ccp(filename='../ccp_IU_HRV.pkl', fmt=None)
10
11# Create spacing
12lats = np.arange(41, 43.5, 0.05)
13lons = np.arange(-72.7, -69.5, 0.05)
14z = np.linspace(-10, 200, 211)
15
16# Plot volume slices: vplot is a VolumePlot object
17vplot = ccpstack.plot_volume_sections(
18 lons, lats, zmax=211, lonsl=-71.45, latsl=42.5, zsl=23)
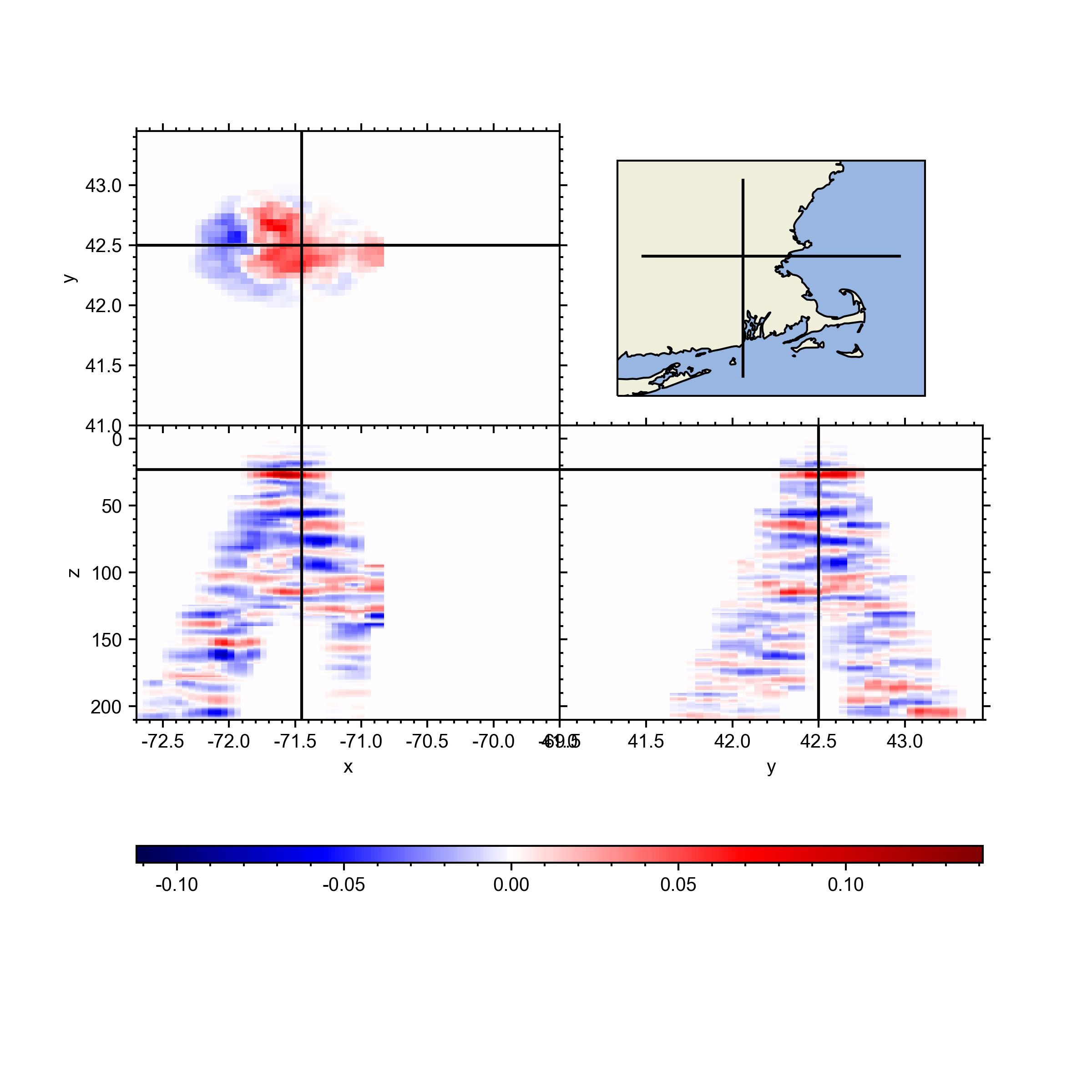
Cross Section#
We can build cross sections with plot_cross_section()
without actually compute a full volume. This is
done (like the computation of the full volume) by using a KDTree that
provides weighted nearest neighbour interpolation on a sphere.
1import numpy as np
2from pyglimer.ccp.ccp import read_ccp
3import matplotlib.pyplot as plt
4
5# Read the CCP Stack
6ccpstack = read_ccp(filename='../ccp_IU_HRV.pkl', fmt=None)
7
8# Create points waypoints for the cross section
9lat0 = np.array([42.5, 42.5])
10lon0 = np.array([-72.7, -69.5])
11lat1 = np.array([41.5, 43.5])
12lon1 = np.array([-71.45, -71.45])
13
14# Set RF boundaries
15mapextent = [-73.5, -69, 41, 44]
16depthextent = [0, 200]
17vmin = -0.1
18vmax = 0.1
19
20# Plot cross sections
21ax1, geoax = ccpstack.plot_cross_section(
22 lat0, lon0, z0=23, vmin=vmin, vmax=vmax,
23 mapplot=True, minillum=1, label="A",
24 depthextent=depthextent,
25 mapextent=mapextent)
26ax2, _ = ccpstack.plot_cross_section(
27 lat1, lon1, vmin=vmin, vmax=vmax,
28 geoax=geoax, mapplot=True,
29 minillum=1, label="B",
30 depthextent=depthextent
31)
32
33plt.show()
This snippet creates a map including the illumination
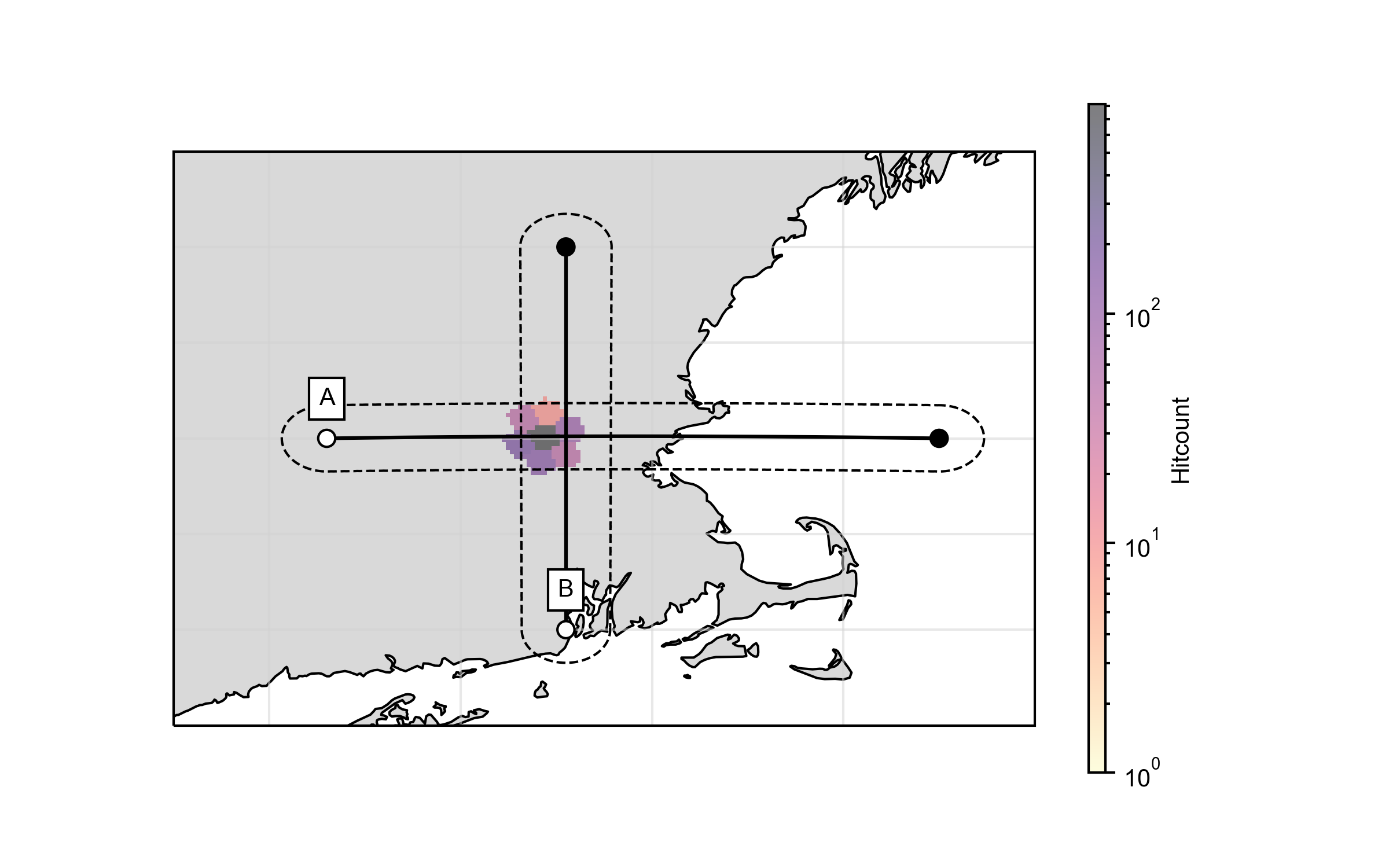
and the respective cross sections A
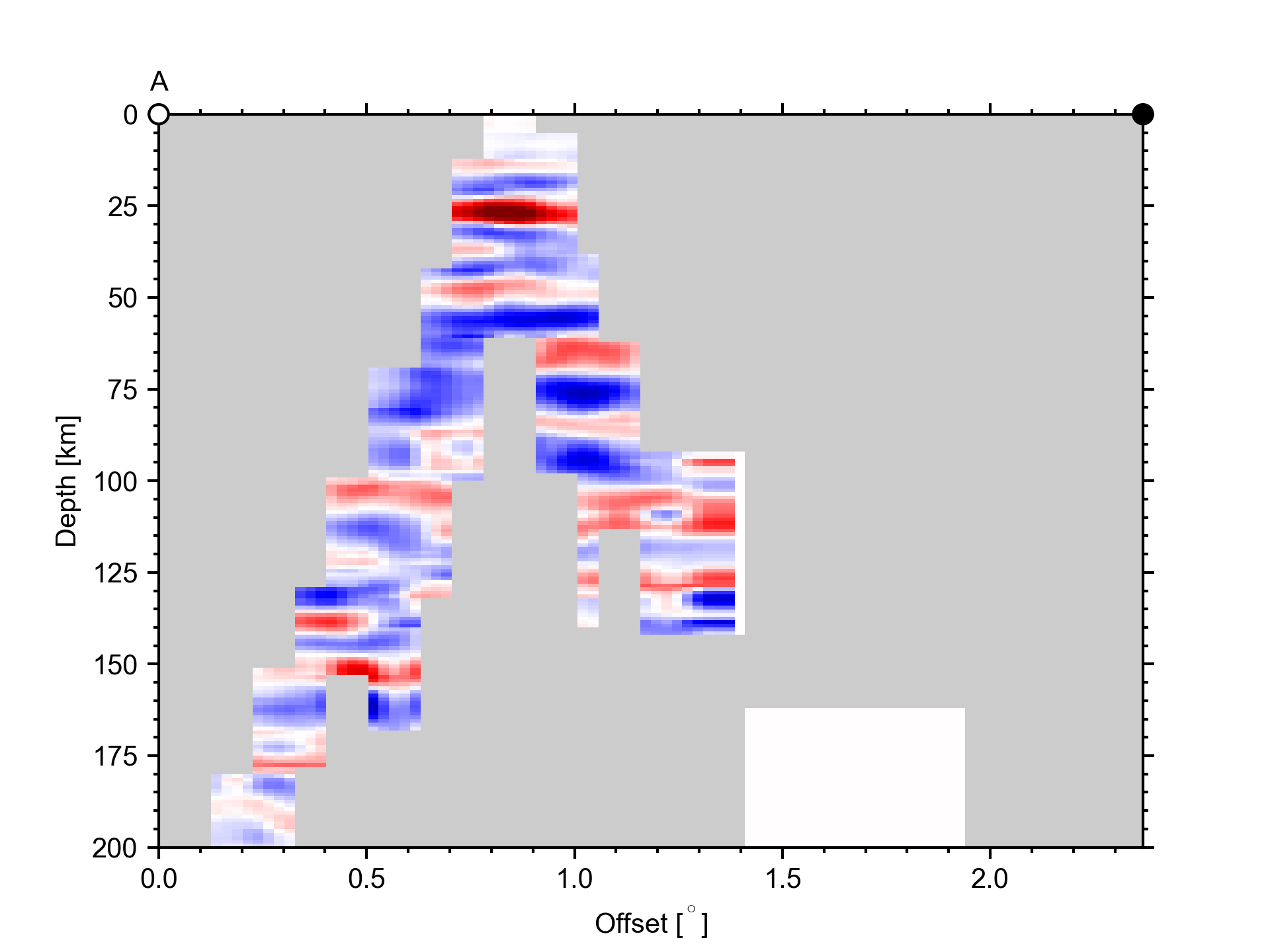
and B
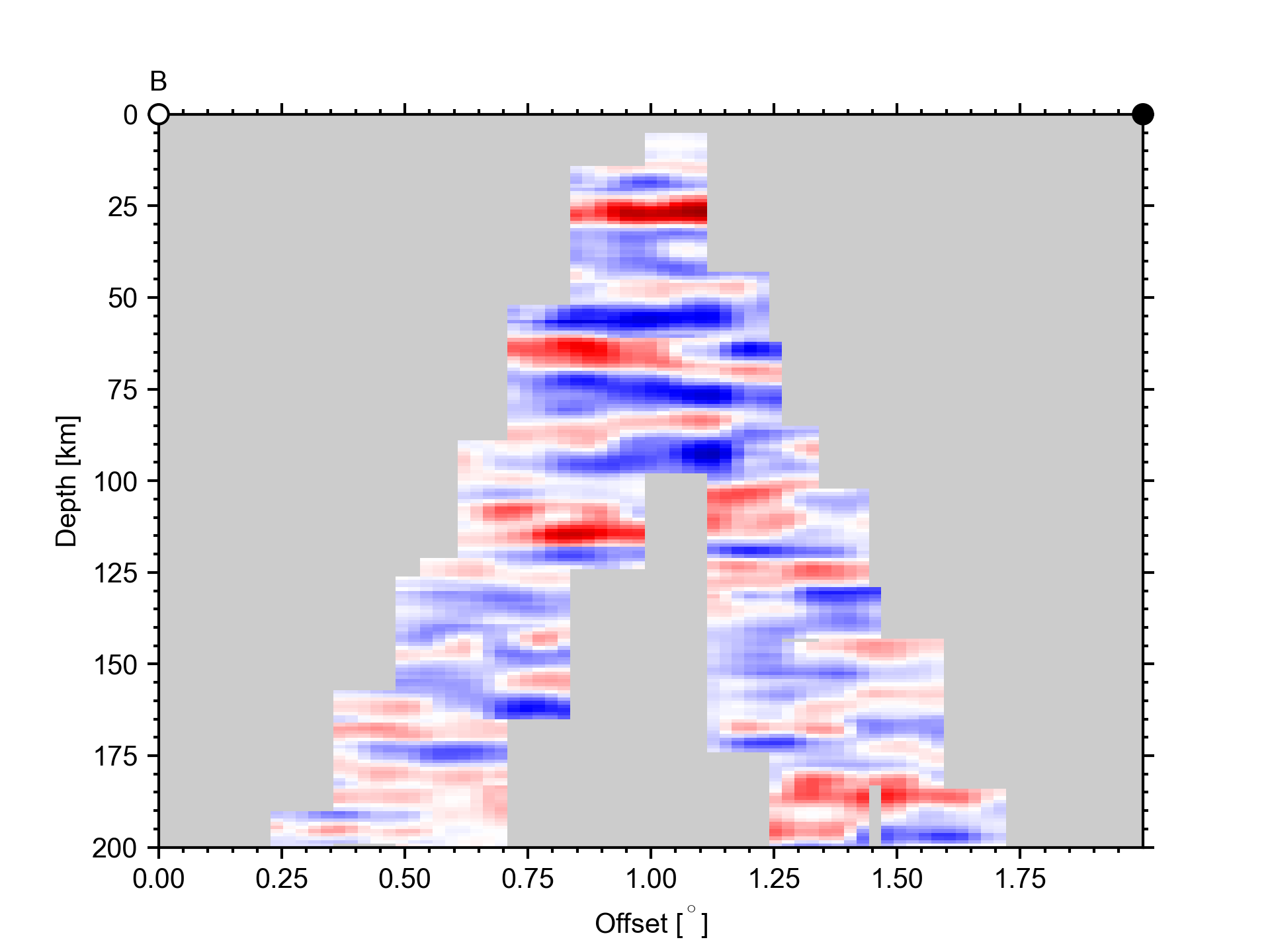
Exploring your CCP-Stack interactively#
You can use explore()
to create an interactive window
that slides through your CCP object.