Note
Go to the end to download the full example code
SAC Database#
In this Tutorial we are going to get all good receiver functions for the year 2018 for station IU-HRV ([Adam Dziewonski Observatory](http://www.seismology.harvard.edu/hrv.html)).
To Compute the receiver function we need to download and organize the observed data. PyGLImER will automatically take care of these things for you in the background, but do check out the ‘Database Docs’ if you aren’t familiar.
Downloading Event & Station Metadata#
To download the data we use the pyglimer.waveform.request.Request
class. The first method from this class that we are going to use is the
download event catalog public method
pyglimer.waveform.request.Request.download_evtcat()
, to get a set
of events that contains all wanted earthquakes. This method is launched
automatically upon initialization.
To initialize said class we setup a parameter dictionary, with all the needed information. Let’s look at the expected information:
# sphinx_gallery_thumbnail_number = 1
# sphinx_gallery_dummy_images = 1
First let’s get a path where to create the data.
# Some needed Imports
import os
from obspy import UTCDateTime
from pyglimer.waveform.request import Request
# Get notebook path for future reference of the database:
try: db_base_path = ipynb_path
except NameError:
try: db_base_path = os.path.dirname(os.path.realpath(__file__))
except NameError: db_base_path = os.getcwd()
# Define file locations
proj_dir = os.path.join(db_base_path, 'tmp', 'database_sac')
request_dict = {
# Necessary arguments
'proj_dir': proj_dir,
'raw_subdir': os.path.join('waveforms', 'raw'),# Directory of the waveforms
'prepro_subdir': os.path.join('waveforms', 'preprocessed'), # Directory of the preprocessed waveforms
'rf_subdir': os.path.join('waveforms', 'RF'), # Directory of the receiver functions
'statloc_subdir': 'stations', # Directory stations
'evt_subdir': 'events', # Directory of the events
'log_subdir': 'log', # Directory for the logs
'loglvl': 'WARNING', # logging level
'format': 'sac', # Format to save database in
"phase": "P", # 'P' or 'S' receiver functions
"rot": "RTZ", # Coordinate system to rotate to
"deconmeth": "waterlevel", # Deconvolution method
"starttime": UTCDateTime(2021, 1, 1, 0, 0, 0), # Starttime of database.
# Here, starttime of HRV
"endtime": UTCDateTime(2021, 7, 1, 0, 0, 0), # Endtimetime of database
# kwargs below
"pol": 'v', # Source wavelet polaristion. Def. "v" --> SV
"minmag": 5.5, # Earthquake minimum magnitude. Def. 5.5
"event_coords": None, # Specific event?. Def. None
"network": "IU", # Restricts networks. Def. None
"station": "HRV", # Restricts stations. Def. None
"waveform_client": ["IRIS"], # FDSN server client (s. obspy). Def. None
"evtcat": None, # If you have already downloaded a set of
# events previously, you can use them here
}
Now that all parameters are in place, let’s initialize the
pyglimer.waveform.request.Request
# Initializing the Request class and downloading the data
R = Request(**request_dict)
The initialization will look for all events for which data is available. To see whether the events make sense we plot a map of the events:
import matplotlib.pyplot as plt
from pyglimer.plot.plot_utils import plot_catalog
from pyglimer.plot.plot_utils import set_mpl_params
# Setting plotting parameters
set_mpl_params()
# Plotting the catalog
plot_catalog(R.evtcat)
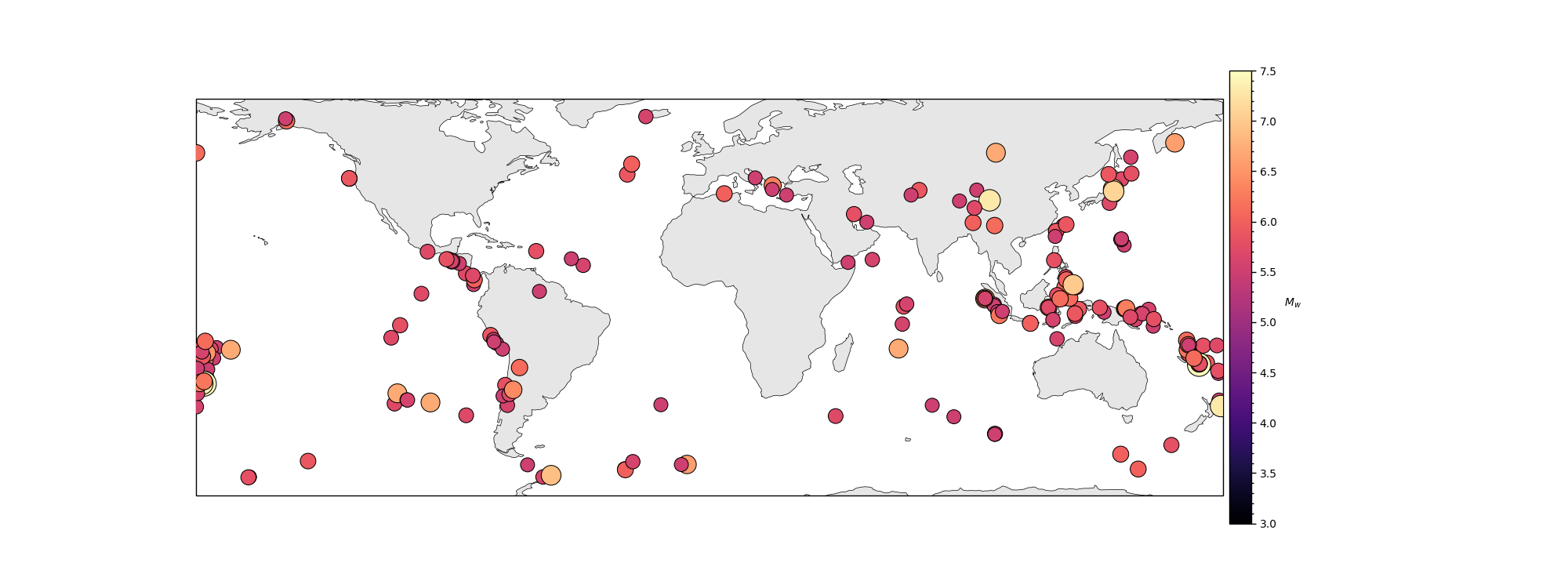
We can also quickly check how many events we gathered.
print(f"There are {len(R.evtcat)} available events")
There are 289 available events
Downloading waveform data and station information#
The next step on our journey to receiver functions is retrieving the corresponding waveform data. To retrieve the waveform data, we can two different public methods of Request: download_waveforms() or, as in this case download_waveforms_small_db().
Both methods use the station and event locations to get viable records of receiver function depending on epicentral distance and traveltimes.
download_waveforms() relies on obspy’s massdownloader and is best suited for extremely large databases (i.e., from many different networks and stations). download_waveforms_small_db() is faster for downloads from few stations and, additionally, has the advantage that the desired networks and stations can be defined as lists and not only as strings. Note that this method requires you to define the channels, you’d like to download (as a string, wildcards allowed).
*NOTE:* This might take a while.
R.download_waveforms_small_db(channel='BH?')
--> Enter TOA event loop for station IU.HRV
Let’s have a quick look at how many miniseeds we actually downloaded.
from glob import glob
# Path to the where the miniseeds are stored
data_storage = os.path.join(
proj_dir, 'waveforms','raw','P','**', '*.mseed')
# Print output
print(f"Number of downloaded waveforms: {len(glob(data_storage))}")
Number of downloaded waveforms: 69
The final step to get you receiver function data is the preprocessing.
Although it is hidden in a single function, which
is pyglimer.waveform.request.Request.preprocess()
A lot of decisions are being made:
Processing steps:
1. Clips waveform to the right length (tz before and ta after theorethical
arrival.)
2. Demean & Detrend
3. Tapering
4. Remove Instrument response, convert to velocity &
simulate havard station
5. Rotation to NEZ and, subsequently, to RTZ.
6. Compute SNR for highpass filtered waveforms (highpass f defined in
qc.lowco) If SNR lower than in qc.SNR_criteria for all filters, rejects
waveform.
7. Write finished and filtered waveforms to folder
specified in qc.outputloc.
8. Write info file with shelf containing station,
event and waveform information.
9. (Optional) If we had chosen a different coordinate system in rot
than RTZ, it would now cast the preprocessed waveforms information
that very coordinate system.
10. Deconvolution with method deconmeth
from our dict is perfomed.
It again uses the request class to perform this. The if __name__ ...
expression is needed for running this examples
R.preprocess(hc_filt=1.5, client='single')
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 35.42it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:51,027 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.77465665 1.00897487 6.76707489]\n [0.78395596 1.00488542 6.3984945 ]\n [1.99114243 1.19502438 6.35761708]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.77465665 1.00897487 6.76707489]\n [0.78395596 1.00488542 6.3984945 ]\n [1.99114243 1.19502438 6.35761708]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 23.87it/s]
100%|##########| 3/3 [00:00<00:00, 23.82it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4332.96it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 5019.11it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4961.72it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 42.92it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:51,157 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.55738492 1.52284439 4.12091759]\n [0.53260342 1.4382248 3.74746545]\n [1.11606256 0.68984621 1.92233572]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.55738492 1.52284439 4.12091759]\n [0.53260342 1.4382248 3.74746545]\n [1.11606256 0.68984621 1.92233572]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 52.88it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:51,214 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.34698491 1.75790696 0.17980923]\n [0.53975226 1.2397252 0.47975826]\n [0.97949665 1.76450105 2.22356795]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.34698491 1.75790696 0.17980923]\n [0.53975226 1.2397252 0.47975826]\n [0.97949665 1.76450105 2.22356795]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
2023-03-29 10:31:51,270 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.09763721 0.86116757 6.57545482]\n [1.63770847 0.79814294 4.98739698]\n [1.84310136 0.74321738 4.36383089]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.09763721 0.86116757 6.57545482]\n [1.63770847 0.79814294 4.98739698]\n [1.84310136 0.74321738 4.36383089]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 26.94it/s]
100%|##########| 3/3 [00:00<00:00, 26.88it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:51,329 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.05215338 0.27042716 0.74857378]\n [1.95892751 0.28213521 0.6040649 ]\n [5.14329143 0.42889155 2.30579555]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.05215338 0.27042716 0.74857378]\n [1.95892751 0.28213521 0.6040649 ]\n [5.14329143 0.42889155 2.30579555]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
2023-03-29 10:31:51,388 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.79974257 0.94303415 1.93664348]\n [0.81125452 0.84643511 1.88741756]\n [3.86171499 0.40865182 7.88765905]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.79974257 0.94303415 1.93664348]\n [0.81125452 0.84643511 1.88741756]\n [3.86171499 0.40865182 7.88765905]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 25.48it/s]
100%|##########| 3/3 [00:00<00:00, 25.43it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:51,447 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.25191365 0.6969515 0.66039297]\n [1.26440898 0.71294255 0.66727628]\n [1.54331557 0.90504796 1.24511069]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.25191365 0.6969515 0.66039297]\n [1.26440898 0.71294255 0.66727628]\n [1.54331557 0.90504796 1.24511069]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 51.11it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:51,506 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.62783222 1.33640018 2.51115809]\n [2.80713403 1.46571748 2.89194389]\n [1.43486858 2.21896923 2.37325583]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.62783222 1.33640018 2.51115809]\n [2.80713403 1.46571748 2.89194389]\n [1.43486858 2.21896923 2.37325583]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 51.47it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4709.17it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4703.89it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:51,564 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.36946357 1.59009554 1.33959493]\n [0.39248076 1.5084356 1.21811842]\n [0.7883569 1.4916343 1.7627449 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.36946357 1.59009554 1.33959493]\n [0.39248076 1.5084356 1.21811842]\n [0.7883569 1.4916343 1.7627449 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 53.71it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:51,623 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[4.6256822 1.26814363 5.60683339]\n [4.11921133 1.05732341 4.87549887]\n [2.24663193 0.80906255 2.756494 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[4.6256822 1.26814363 5.60683339]\n [4.11921133 1.05732341 4.87549887]\n [2.24663193 0.80906255 2.756494 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
2023-03-29 10:31:51,688 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.18174212 1.9058565 5.84034768]\n [ 1.15656671 1.92210902 5.17544844]\n [ 2.25286359 0.76143188 13.24849562]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.18174212 1.9058565 5.84034768]\n [ 1.15656671 1.92210902 5.17544844]\n [ 2.25286359 0.76143188 13.24849562]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
67%|######6 | 2/3 [00:00<00:00, 16.32it/s]
100%|##########| 3/3 [00:00<00:00, 24.36it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:51,745 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 6.33644578 0.92816468 22.16567589]\n [ 6.45708456 0.91802692 20.92932409]\n [ 4.57169208 0.59941267 12.87209401]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 6.33644578 0.92816468 22.16567589]\n [ 6.45708456 0.91802692 20.92932409]\n [ 4.57169208 0.59941267 12.87209401]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 53.39it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:51,803 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[12.31516683 0.52109744 3.62905983]\n [10.17945695 0.54410724 2.42748922]\n [ 2.27777848 3.66156507 2.98023857]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[12.31516683 0.52109744 3.62905983]\n [10.17945695 0.54410724 2.42748922]\n [ 2.27777848 3.66156507 2.98023857]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
2023-03-29 10:31:51,859 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.79234939 1.31997455 0.79656996]\n [0.8709967 1.31212136 1.15438836]\n [0.79439029 1.95865842 2.88900837]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.79234939 1.31997455 0.79656996]\n [0.8709967 1.31212136 1.15438836]\n [0.79439029 1.95865842 2.88900837]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 26.34it/s]
100%|##########| 3/3 [00:00<00:00, 26.28it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:51,919 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[4.86963346 0.49996072 2.28061054]\n [4.42374213 0.412849 2.02334711]\n [1.56623265 0.86088051 1.33365883]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[4.86963346 0.49996072 2.28061054]\n [4.42374213 0.412849 2.02334711]\n [1.56623265 0.86088051 1.33365883]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
2023-03-29 10:31:51,977 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.207504 3.91981655 0.39421082]\n [0.21870725 3.37556495 0.34303395]\n [1.03521629 0.86202356 0.63562027]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.207504 3.91981655 0.39421082]\n [0.21870725 3.37556495 0.34303395]\n [1.03521629 0.86202356 0.63562027]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 25.69it/s]
100%|##########| 3/3 [00:00<00:00, 25.64it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4096.00it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,037 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.93197151 1.25958794 1.72720219]\n [1.70396234 1.41538054 1.54498997]\n [3.18254879 0.86279844 6.14538468]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.93197151 1.25958794 1.72720219]\n [1.70396234 1.41538054 1.54498997]\n [3.18254879 0.86279844 6.14538468]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 51.24it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,097 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.19458544 0.81832005 2.47912244]\n [ 1.29881621 0.9082169 2.35966301]\n [ 5.94398005 0.36718399 10.02892903]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.19458544 0.81832005 2.47912244]\n [ 1.29881621 0.9082169 2.35966301]\n [ 5.94398005 0.36718399 10.02892903]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 50.56it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,221 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.97016363 1.16794642 16.36215449]\n [ 1.07574577 1.54145175 7.85548671]\n [ 1.08863403 0.95467032 4.94234647]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.97016363 1.16794642 16.36215449]\n [ 1.07574577 1.54145175 7.85548671]\n [ 1.08863403 0.95467032 4.94234647]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 24.29it/s]
100%|##########| 3/3 [00:00<00:00, 24.24it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4191.51it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,281 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.75149505 0.71290573 3.01690911]\n [ 1.73201783 0.71279446 2.9250638 ]\n [ 2.30373545 0.30048345 10.23488144]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.75149505 0.71290573 3.01690911]\n [ 1.73201783 0.71279446 2.9250638 ]\n [ 2.30373545 0.30048345 10.23488144]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 50.81it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4695.12it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,343 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.23852881 5.35085842 0.86698052]\n [0.19491652 6.00248374 0.90349542]\n [0.61894996 3.39331302 1.9376621 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.23852881 5.35085842 0.86698052]\n [0.19491652 6.00248374 0.90349542]\n [0.61894996 3.39331302 1.9376621 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 50.46it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,401 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.3058556 0.36227009 2.46243893]\n [1.23795793 0.39560712 2.42969189]\n [0.71088007 0.77127428 5.8917886 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.3058556 0.36227009 2.46243893]\n [1.23795793 0.39560712 2.42969189]\n [0.71088007 0.77127428 5.8917886 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 52.68it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4078.74it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,467 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.37695608 0.61037371 1.45286415]\n [0.35745489 0.61754662 1.35660595]\n [0.7081454 0.67376546 1.03013349]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.37695608 0.61037371 1.45286415]\n [0.35745489 0.61754662 1.35660595]\n [0.7081454 0.67376546 1.03013349]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 45.66it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,527 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.48765892 1.52089774 0.36097947]\n [0.40434299 1.75794151 0.35558971]\n [1.11930979 1.38768576 1.1200572 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.48765892 1.52089774 0.36097947]\n [0.40434299 1.75794151 0.35558971]\n [1.11930979 1.38768576 1.1200572 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 51.10it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4436.85it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,586 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.50137621 8.08273098 1.81695077]\n [0.49684474 8.11940006 1.86362684]\n [2.74568051 1.72460876 8.4104976 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.50137621 8.08273098 1.81695077]\n [0.49684474 8.11940006 1.86362684]\n [2.74568051 1.72460876 8.4104976 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 51.90it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4771.68it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4602.38it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4967.59it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,647 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.11852153 0.20478462 1.36658408]\n [1.9033695 0.19330488 1.29824582]\n [0.81758287 1.31993168 1.79033817]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.11852153 0.20478462 1.36658408]\n [1.9033695 0.19330488 1.29824582]\n [0.81758287 1.31993168 1.79033817]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
2023-03-29 10:31:52,712 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[3.14417656 0.21762841 1.18374098]\n [3.22423182 0.22454063 1.1434097 ]\n [0.71133617 1.17235395 1.40356982]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[3.14417656 0.21762841 1.18374098]\n [3.22423182 0.22454063 1.1434097 ]\n [0.71133617 1.17235395 1.40356982]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 24.69it/s]
100%|##########| 3/3 [00:00<00:00, 24.64it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,770 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.30474186 1.65675168 2.33766345]\n [1.31324529 1.72973074 2.24288312]\n [2.23032937 0.8877333 3.31340704]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.30474186 1.65675168 2.33766345]\n [1.31324529 1.72973074 2.24288312]\n [2.23032937 0.8877333 3.31340704]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 51.69it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4604.07it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,828 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.48713345 1.26278022 10.42521761]\n [ 0.57210968 2.18397877 3.08139534]\n [ 1.82669697 1.25985703 5.25424248]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.48713345 1.26278022 10.42521761]\n [ 0.57210968 2.18397877 3.08139534]\n [ 1.82669697 1.25985703 5.25424248]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 53.97it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,887 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 0.31699098 10.72221528 1.1373009 ]\n [ 0.3311078 10.10227358 1.12144035]\n [ 1.11756988 1.31805085 1.84244938]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 0.31699098 10.72221528 1.1373009 ]\n [ 0.3311078 10.10227358 1.12144035]\n [ 1.11756988 1.31805085 1.84244938]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 51.53it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:52,947 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.82846419 0.3146184 3.86160363]\n [0.85182855 0.30010691 3.81857979]\n [0.99119347 0.64149876 2.79141971]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.82846419 0.3146184 3.86160363]\n [0.85182855 0.30010691 3.81857979]\n [0.99119347 0.64149876 2.79141971]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 49.69it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,005 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 2.33415618 0.54938196 2.80578659]\n [ 2.99452423 0.56630551 2.62776272]\n [ 5.22110221 1.13268981 16.04885634]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 2.33415618 0.54938196 2.80578659]\n [ 2.99452423 0.56630551 2.62776272]\n [ 5.22110221 1.13268981 16.04885634]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
2023-03-29 10:31:53,061 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.19066722 1.02539295 0.32687827]\n [0.20092153 0.99037003 0.32560234]\n [0.70024576 1.03154053 1.82284694]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.19066722 1.02539295 0.32687827]\n [0.20092153 0.99037003 0.32560234]\n [0.70024576 1.03154053 1.82284694]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 26.47it/s]
100%|##########| 3/3 [00:00<00:00, 26.42it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,122 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.5134158 7.12916547 4.34975005]\n [ 1.21763932 5.87758287 3.46152152]\n [ 6.55444519 1.95193533 24.43550174]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.5134158 7.12916547 4.34975005]\n [ 1.21763932 5.87758287 3.46152152]\n [ 6.55444519 1.95193533 24.43550174]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 49.52it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4582.27it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,186 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.53211509 0.58742688 2.16797096]\n [1.52884941 0.57955025 2.09763533]\n [0.7714919 1.17997521 1.25198413]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.53211509 0.58742688 2.16797096]\n [1.52884941 0.57955025 2.09763533]\n [0.7714919 1.17997521 1.25198413]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 47.77it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4648.29it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4959.76it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4832.15it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 5047.30it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4928.68it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4940.29it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4930.61it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 5023.12it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 5015.11it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4913.28it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4928.68it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4832.15it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4975.45it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4981.36it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4951.95it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4944.17it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 5023.12it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,266 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.11587664 1.0889175 0.66435922]\n [0.8559692 0.9865344 0.51811433]\n [0.73876595 1.99303477 1.02615493]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.11587664 1.0889175 0.66435922]\n [0.8559692 0.9865344 0.51811433]\n [0.73876595 1.99303477 1.02615493]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
2023-03-29 10:31:53,322 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.3572727 1.27520298 1.04087958]\n [1.31233243 0.67306272 0.61712604]\n [2.90442703 0.96157888 2.37805971]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.3572727 1.27520298 1.04087958]\n [1.31233243 0.67306272 0.61712604]\n [2.90442703 0.96157888 2.37805971]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
67%|######6 | 2/3 [00:00<00:00, 16.99it/s]
100%|##########| 3/3 [00:00<00:00, 25.36it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4624.37it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,380 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.99901602 0.70355602 4.24354721]\n [2.46879809 0.87875136 3.65038726]\n [3.44821161 0.41931474 5.83365682]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.99901602 0.70355602 4.24354721]\n [2.46879809 0.87875136 3.65038726]\n [3.44821161 0.41931474 5.83365682]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
2023-03-29 10:31:53,436 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.94202712 4.1720967 1.7250499 ]\n [0.885124 4.61889442 1.7591223 ]\n [1.27150042 0.63014472 3.96690695]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.94202712 4.1720967 1.7250499 ]\n [0.885124 4.61889442 1.7591223 ]\n [1.27150042 0.63014472 3.96690695]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
67%|######6 | 2/3 [00:00<00:00, 17.88it/s]
100%|##########| 3/3 [00:00<00:00, 26.67it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,493 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.56476423 0.49393762 4.17525705]\n [1.29827061 0.60459027 3.93034809]\n [0.91875839 1.45908496 2.44306798]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.56476423 0.49393762 4.17525705]\n [1.29827061 0.60459027 3.93034809]\n [0.91875839 1.45908496 2.44306798]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 53.98it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4159.64it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,553 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.46355877 2.07757762 0.24869133]\n [0.50323266 1.79471544 0.27856967]\n [0.98619123 0.91055669 1.96065821]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.46355877 2.07757762 0.24869133]\n [0.50323266 1.79471544 0.27856967]\n [0.98619123 0.91055669 1.96065821]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 51.22it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,609 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.18765612 1.59511094 0.77840285]\n [0.15629658 1.97458159 0.79474687]\n [0.93388211 0.68341089 3.51859875]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.18765612 1.59511094 0.77840285]\n [0.15629658 1.97458159 0.79474687]\n [0.93388211 0.68341089 3.51859875]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 53.50it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,669 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.47122283 1.33198142 7.56635662]\n [1.4804066 1.2939493 7.53332819]\n [1.46198128 0.24910905 3.23563016]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.47122283 1.33198142 7.56635662]\n [1.4804066 1.2939493 7.53332819]\n [1.46198128 0.24910905 3.23563016]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 51.09it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 3978.16it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,727 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.32042807 3.03786004 7.63596366]\n [ 1.25786885 1.07473834 8.32546965]\n [ 9.05911085 2.58990346 81.35894004]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 1.32042807 3.03786004 7.63596366]\n [ 1.25786885 1.07473834 8.32546965]\n [ 9.05911085 2.58990346 81.35894004]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 52.72it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,784 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.70015226 0.24010874 2.2959211 ]\n [1.78360645 0.21788584 2.59740144]\n [1.05162744 1.76552374 5.8017984 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.70015226 0.24010874 2.2959211 ]\n [1.78360645 0.21788584 2.59740144]\n [1.05162744 1.76552374 5.8017984 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 53.53it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4753.65it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4913.28it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4934.48it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4516.48it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 5045.27it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4741.11it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,847 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.96067918 1.48343149 2.7944811 ]\n [2.73502545 1.30625742 2.45446028]\n [0.26024097 1.24864774 3.27127154]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.96067918 1.48343149 2.7944811 ]\n [2.73502545 1.30625742 2.45446028]\n [0.26024097 1.24864774 3.27127154]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 53.29it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4718.00it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:53,904 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[3.68602203 0.49919763 0.82143315]\n [3.67648557 0.51242735 0.83682993]\n [1.93360987 1.186115 1.35390583]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[3.68602203 0.49919763 0.82143315]\n [3.67648557 0.51242735 0.83682993]\n [1.93360987 1.186115 1.35390583]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 53.68it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 3919.91it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:54,035 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.39436265 1.06161047 0.80833058]\n [0.41064204 0.92787455 0.80650057]\n [1.15155032 0.8069674 1.41004968]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.39436265 1.06161047 0.80833058]\n [0.41064204 0.92787455 0.80650057]\n [1.15155032 0.8069674 1.41004968]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 23.34it/s]
100%|##########| 3/3 [00:00<00:00, 23.30it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:54,091 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.4540302 1.66351048 0.93836724]\n [0.46214179 1.71081926 0.87659122]\n [2.1941202 0.33036774 1.50860983]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.4540302 1.66351048 0.93836724]\n [0.46214179 1.71081926 0.87659122]\n [2.1941202 0.33036774 1.50860983]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 53.45it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:54,150 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.18428771 0.7890499 1.66870549]\n [1.30185146 2.13476244 1.99134201]\n [2.06818988 0.69539516 4.69082092]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[2.18428771 0.7890499 1.66870549]\n [1.30185146 2.13476244 1.99134201]\n [2.06818988 0.69539516 4.69082092]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 51.60it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4955.85it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:54,210 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.4696332 0.3180262 3.43923645]\n [1.26079935 0.2926006 3.74712701]\n [0.66141491 0.87704583 1.53416411]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.4696332 0.3180262 3.43923645]\n [1.26079935 0.2926006 3.74712701]\n [0.66141491 0.87704583 1.53416411]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
2023-03-29 10:31:54,266 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.96114395 1.31404547 0.58601326]\n [0.96214625 1.35656142 0.59997236]\n [1.08262967 0.5134989 1.09100031]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.96114395 1.31404547 0.58601326]\n [0.96214625 1.35656142 0.59997236]\n [1.08262967 0.5134989 1.09100031]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
67%|######6 | 2/3 [00:00<00:00, 17.57it/s]2023-03-29 10:31:54,334 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 2.69179137 0.39428315 16.48242578]\n [ 2.07954956 0.60278277 13.03671662]\n [ 7.48471173 1.44069323 36.45517572]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 2.69179137 0.39428315 16.48242578]\n [ 2.07954956 0.60278277 13.03671662]\n [ 7.48471173 1.44069323 36.45517572]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 16.45it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:54,393 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.92829267 0.88957341 0.47600718]\n [0.96142953 0.81388053 0.50033741]\n [1.01812335 1.40434638 2.15255299]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.92829267 0.88957341 0.47600718]\n [0.96142953 0.81388053 0.50033741]\n [1.01812335 1.40434638 2.15255299]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
2023-03-29 10:31:54,451 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.18599079 0.96763175 1.57652515]\n [1.1402394 0.92690482 1.13532165]\n [1.1624181 1.54171883 0.57884433]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.18599079 0.96763175 1.57652515]\n [1.1402394 0.92690482 1.13532165]\n [1.1624181 1.54171883 0.57884433]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
67%|######6 | 2/3 [00:00<00:00, 17.21it/s]2023-03-29 10:31:54,509 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 3.02557643 1.85270331 3.39576384]\n [ 3.30553404 1.8631756 2.9657923 ]\n [13.28056921 1.44392776 8.33493155]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 3.02557643 1.85270331 3.39576384]\n [ 3.30553404 1.8631756 2.9657923 ]\n [13.28056921 1.44392776 8.33493155]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 17.18it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:54,568 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.04715985 1.23905155 0.86147272]\n [1.15440674 1.00576103 0.89681645]\n [0.61940983 1.08995289 1.12565782]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.04715985 1.23905155 0.86147272]\n [1.15440674 1.00576103 0.89681645]\n [0.61940983 1.08995289 1.12565782]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 51.12it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:54,704 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.08074301 0.40361461 0.2492072 ]\n [0.06956622 1.92427215 0.67380438]\n [3.21690923 0.43727423 0.6300436 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.08074301 0.40361461 0.2492072 ]\n [0.06956622 1.92427215 0.67380438]\n [3.21690923 0.43727423 0.6300436 ]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 22.29it/s]
100%|##########| 3/3 [00:00<00:00, 22.25it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:54,763 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.98482589 0.06870258 0.53173313]\n [1.93330114 0.06596743 0.6224342 ]\n [0.47420229 1.4134802 0.48149862]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[1.98482589 0.06870258 0.53173313]\n [1.93330114 0.06596743 0.6224342 ]\n [0.47420229 1.4134802 0.48149862]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
2023-03-29 10:31:54,825 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 2.30954048 1.50889535 27.06374045]\n [ 0.83829111 4.06996713 15.10360683]\n [ 3.49074656 10.37422203 24.08254121]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[ 2.30954048 1.50889535 27.06374045]\n [ 0.83829111 4.06996713 15.10360683]\n [ 3.49074656 10.37422203 24.08254121]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
67%|######6 | 2/3 [00:00<00:00, 16.58it/s]
100%|##########| 3/3 [00:00<00:00, 24.75it/s]
0%| | 0/3 [00:00<?, ?it/s]2023-03-29 10:31:54,883 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.97530422 1.6348726 0.96184111]\n [1.0147696 1.3706552 0.78644021]\n [1.56252016 0.77566709 0.43324109]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[0.97530422 1.6348726 0.96184111]\n [1.0147696 1.3706552 0.78644021]\n [1.56252016 0.77566709 0.43324109]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 3/3 [00:00<00:00, 52.65it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 41.94it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 4516.48it/s]
0%| | 0/3 [00:00<?, ?it/s]
100%|##########| 3/3 [00:00<00:00, 5041.23it/s]
0%| | 0/1 [00:00<?, ?it/s]2023-03-29 10:31:55,016 - ERROR - ['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[4.51160428 1.85321184 2.87863976]\n [4.92779428 1.8120025 3.22000192]\n [2.68676592 1.04404429 3.41115888]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
ERROR:pyglimer.request.preprocess:['IU.HRV.mseed', TypeError("write_info() missing 1 required positional argument: 'preproloc'")]
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 392, in __waveform_loop
st, crit, infodict = __rotate_qc(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 666, in __rotate_qc
raise SNRError('QC rejected %s' % np.array2string(noisemat))
pyglimer.waveform.preprocess.SNRError: 'QC rejected [[4.51160428 1.85321184 2.87863976]\n [4.92779428 1.8120025 3.22000192]\n [2.68676592 1.04404429 3.41115888]]'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 290, in __event_loop
info = __waveform_loop(
File "/home/runner/work/PyGLImER/PyGLImER/src/pyglimer/waveform/preprocess.py", line 421, in __waveform_loop
write_info(network, station, infodict)
TypeError: write_info() missing 1 required positional argument: 'preproloc'
100%|##########| 1/1 [00:00<00:00, 17.08it/s]
First Receiver functions#
The following few section show how to plot
Single raw RFs
A set of raw RFs
A move-out corrected RF
A set of move-out corrected RFs
Read the IU-HRV receiver functions as a Receiver function stream#
Let’s read a receiver function set and see what it’s all about! (i.e. let’s look at what data a Receiver function trace contains and how we can use it!)
from pyglimer.rf.create import read_rf
path_to_rf = os.path.join(proj_dir, 'waveforms','RF','P','IU','HRV','*.sac')
rfstream = read_rf(path_to_rf)
print(f"Number of RFs: {len(rfstream)}")
Number of RFs: 7
PyGLImER is based on Obspy, but to handle RFs we need some more attributes:
from pprint import pprint
rftrace = rfstream[0]
pprint(rftrace.stats)
Stats({'sampling_rate': 10.0, 'delta': 0.1, 'starttime': UTCDateTime(2021, 1, 10, 4, 4, 10, 319538), 'endtime': UTCDateTime(2021, 1, 10, 4, 6, 40, 219538), 'npts': 1500, 'calib': 1.0, 'network': 'IU', 'station': 'HRV', 'location': '00', 'channel': 'PRF', 'sac': AttribDict({'delta': 0.1, 'depmin': -0.49422535, 'depmax': 0.63900816, 'scale': 1.0, 'b': 0.000538, 'e': 149.90054, 'o': -595.836, 'a': 29.97507, 'stla': 42.5064, 'stlo': -71.5583, 'stel': 200.0, 'evla': -24.0412, 'evlo': -66.5729, 'evdp': 217000.0, 'mag': 6.1, 'user1': 6.337349, 'baz': 175.01988, 'gcarc': 66.419815, 'depmen': 3.451596e-09, 'nzyear': 2021, 'nzjday': 10, 'nzhour': 4, 'nzmin': 4, 'nzsec': 10, 'nzmsec': 319, 'nvhdr': 6, 'npts': 1500, 'iftype': 1, 'iztype': 9, 'leven': 1, 'lpspol': 1, 'lovrok': 1, 'lcalda': 0, 'kstnm': 'HRV', 'khole': '00', 'kuser0': 'time', 'kuser1': 'P', 'kcmpnm': 'PRF', 'knetwk': 'IU'}), '_format': 'SAC', 'station_latitude': 42.5064, 'station_longitude': -71.5583, 'station_elevation': 200.0, 'event_latitude': -24.0412, 'event_longitude': -66.5729, 'event_depth': 217000.0, 'event_magnitude': 6.1, 'event_time': UTCDateTime(2021, 1, 10, 3, 54, 14, 483001), 'onset': UTCDateTime(2021, 1, 10, 4, 4, 40, 294071), 'type': 'time', 'phase': 'P', 'distance': 66.419815, 'back_azimuth': 175.01988, 'slowness': 6.337349})
First Receiver function plots#
If the Receiver functions haven’t been further processed, they are plotted as a function of time. A single receiver function in the stream will be plotted as function of time only. A full stream can make use of the distance measure saved in the sac-header and plot an entire section as a function of time and epicentral distance.
Plot single RF#
Below we show how to plot the receiver function as a function of time, and the clean option, which plots the receiver function without any axes or text.
from pyglimer.plot.plot_utils import set_mpl_params
# Plot RF
rftrace.plot()
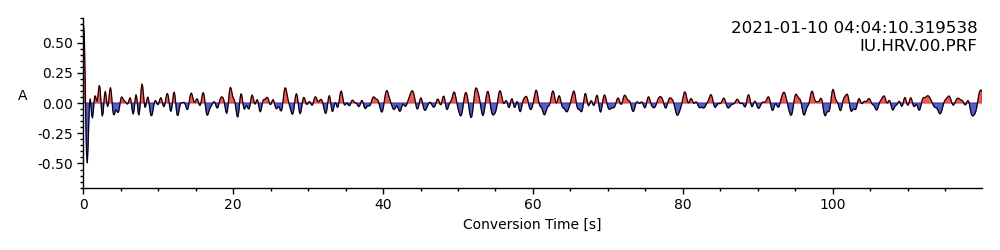
<AxesSubplot: xlabel='Conversion Time [s]', ylabel='A '>
Let’s zoom into the first 20 seconds (~200km)
rftrace.plot(lim=[0,20])
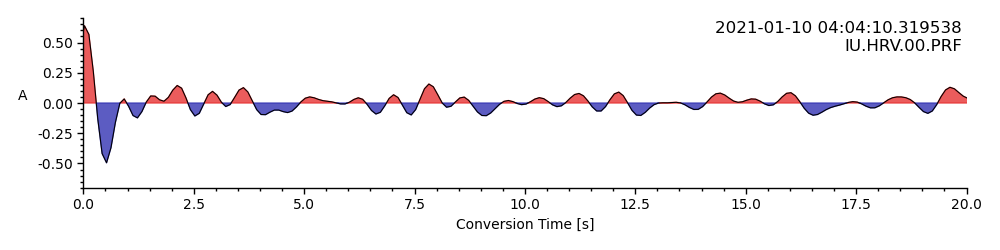
<AxesSubplot: xlabel='Conversion Time [s]', ylabel='A '>
Plot RF section#
Since we have an entire stream of receiver functions at hand, we can plot a section
rfstream.plot(scalingfactor=1)
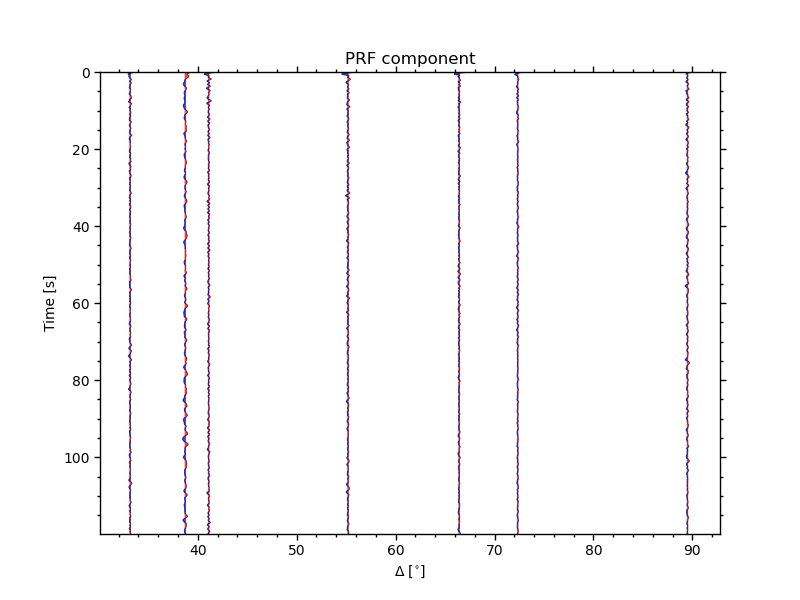
<AxesSubplot: title={'center': 'PRF component'}, xlabel='$\\Delta$ [$^{\\circ}$]', ylabel='Time [s]'>
Similar to the single RF plot we can provide time and epicentral distance limits:
timelimits = (0, 20) # seconds
epilimits = (32, 36) # epicentral distance
rfstream.plot(
scalingfactor=0.25, lim=timelimits, epilimits=epilimits,
linewidth=0.75)
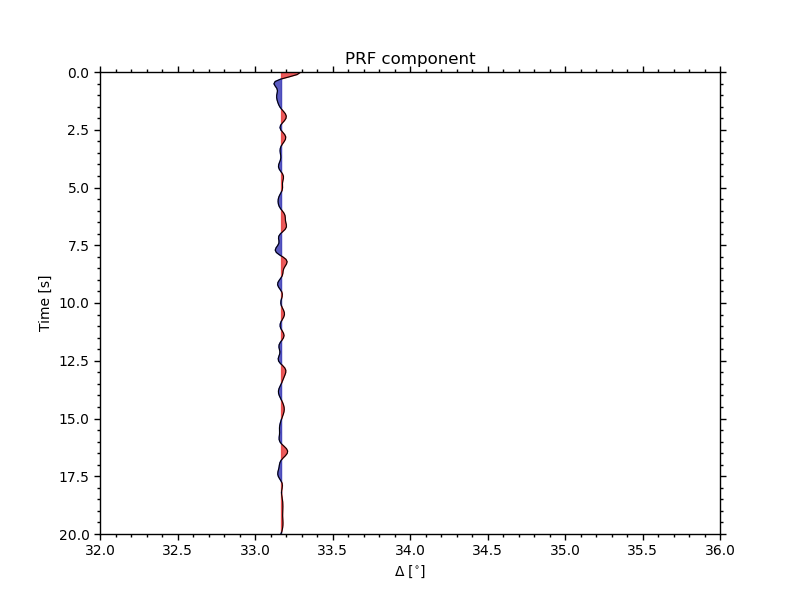
<AxesSubplot: title={'center': 'PRF component'}, xlabel='$\\Delta$ [$^{\\circ}$]', ylabel='Time [s]'>
By increasing the scaling factor and removing the plotted lines, we can already see trends:
rfstream.plot(
scalingfactor=0.5, lim=timelimits, epilimits=epilimits,
line=False)
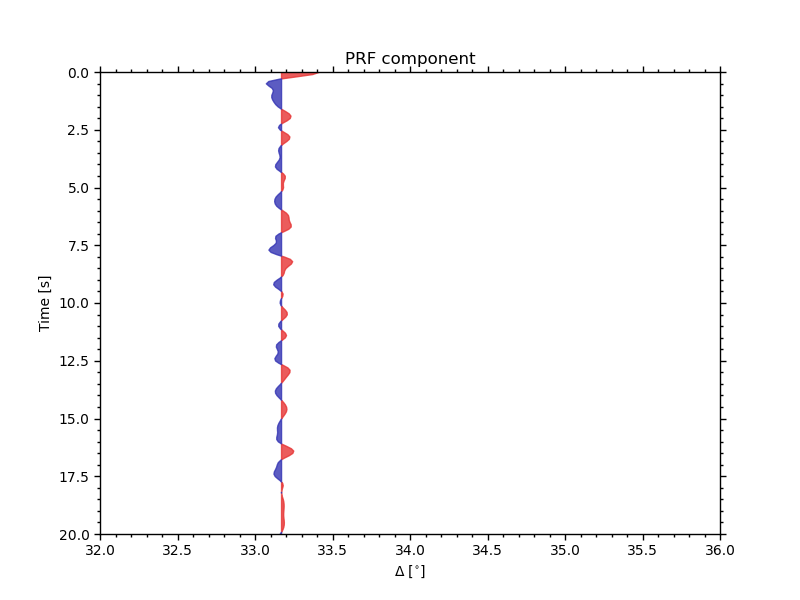
<AxesSubplot: title={'center': 'PRF component'}, xlabel='$\\Delta$ [$^{\\circ}$]', ylabel='Time [s]'>
As simple as that you can create your own receiver functions with just a single smalle script.
Total running time of the script: ( 1 minutes 12.934 seconds)